Features#
In this document we will explain the different features that you can extract from the displacmenet and velocity traces.
Let us first download some demo data that we can use as an illustrative example
from pathlib import Path
import matplotlib.pyplot as plt # For plotting
import mps # Package to load data
import mps_motion # Package for motion analysis
import ap_features as apf # Package for analzying traces
import numpy as np
import logging
# Set log level to warning so that we don't spill to much output
mps_motion.set_log_level(logging.WARNING)
path = Path("data.npy")
if not path.is_file():
mps_motion.utils.download_demo_data(path)
Downloading data. Please wait...
Done downloading data
data = mps.MPS(path)
And let us compute a velocity and displacement trace. First we create an optical flow object
opt_flow = mps_motion.OpticalFlow(data, flow_algorithm="farneback")
Then we compute the velocity
spacing = 5
V = opt_flow.get_velocities(spacing=spacing)
and the mean velocity norm
v_mean_norm = V.norm().mean().compute() * 1000.0 # Multiply by 1000 to convert velocity from um / ms to um / s
And now we estimate the reference frame
reference_frame_index = mps_motion.motion_tracking.estimate_referece_image_from_velocity(
t=data.time_stamps[:-5],
v=v_mean_norm,
)
reference_frame = data.time_stamps[reference_frame_index]
And finally compute the displacement
U = opt_flow.get_displacements(reference_frame=reference_frame)
and compute the mean displacement norm
u_mean_norm = U.norm().mean().compute()
Let us now plot the two traces
fig, ax = plt.subplots(2, 1)
ax[0].plot(data.time_stamps, u_mean_norm)
ax[1].plot(data.time_stamps[:-5], v_mean_norm)
plt.show()
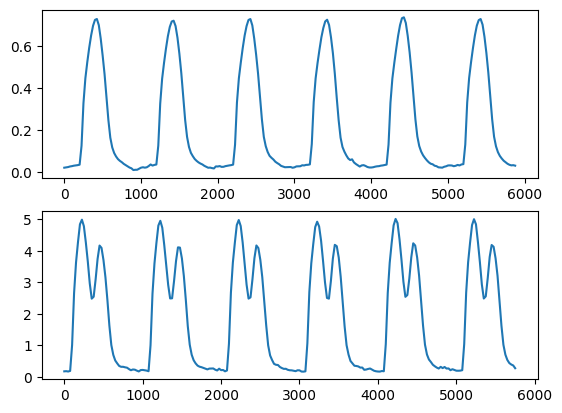
Let us extract the first beat and plot the different features
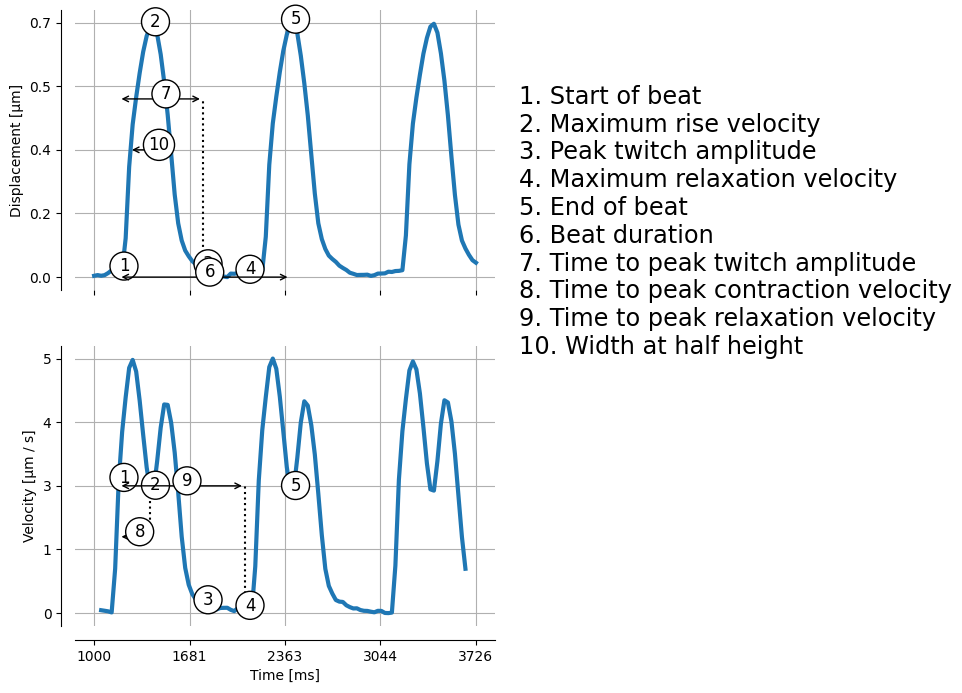
for k, v in mps_motion.stats.compute_features(u=u.y, v=v.y, t=u.t).items():
print(f"{k}: {v[0]}")
Maximum rise velocity: 4.803749099641321
Peak twitch amplitude: 0.6970655570949165
Maximum relaxation velocity: 4.0202496092336055
Beat duration: 925.2019042967277
Time to peak twitch amplitude: 425.0717773436463
Time to peak contraction velocity: 225.09082031251637
Time to peak relaxation velocity: 450.0788574218859
Width at half height: 290.5939363753341